Using ACF Pro Section Styles with Flexible Content as a Page Builder
Using the Flexible Content field from Advanced Custom Fields allows you to quickly set up a page builder for your theme. However, this method lacks control over the appearance of specific sections. To implement this you would typically need to add fields for margins, borders, padding, and background for each layout you create for your Flexible Content. This ends up being repetitive and unintuitive for users.
To help with this I’ve created the ACF Section Styles plugin, which adds a new field type specifically for configuring styles:
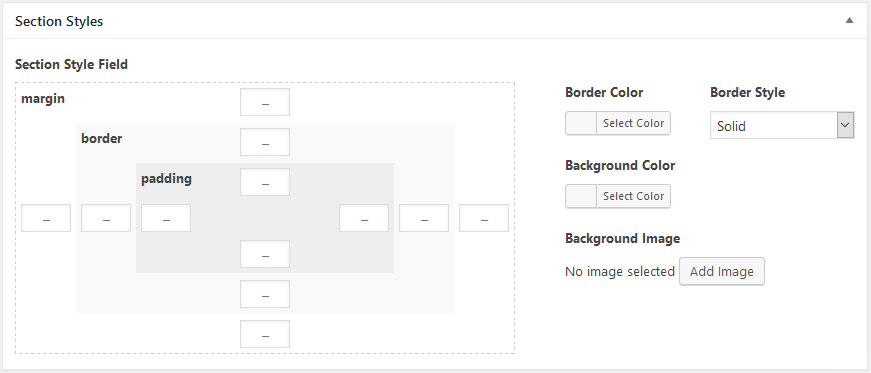
This field provides an array of values for the margin, border, padding, and background styles, but you will still need to apply the styles using your preferred method. A sample method using the wp_add_inline_style
function is demonstrated below.
Create your Flexible Content Field
In the ACF Custom Fields section a new group is created along with a Flexible Content field called content_sections
. A layout is created called default
that contains a content area WYSIWYG, as well as a Section Style field:
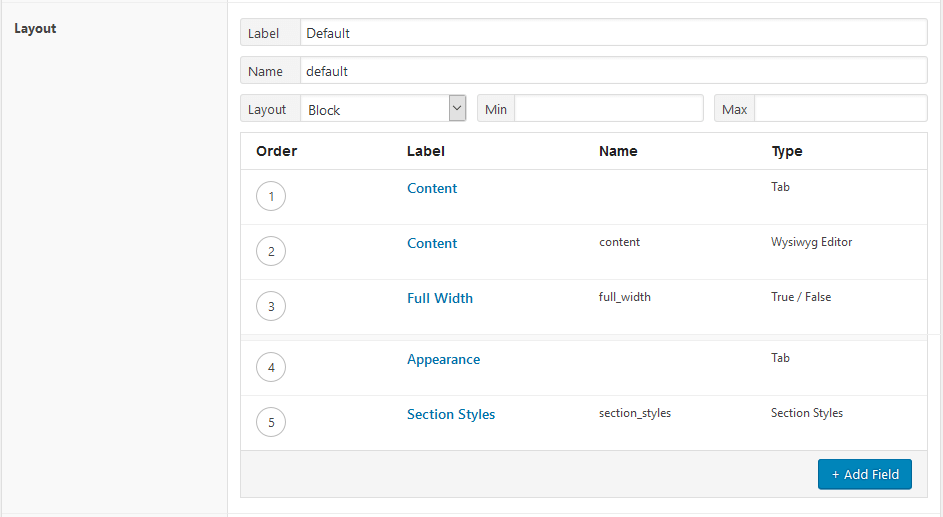
Add Your Content
Now when editing a page or post that uses your new Flexible Content field you’ll see something like the following:
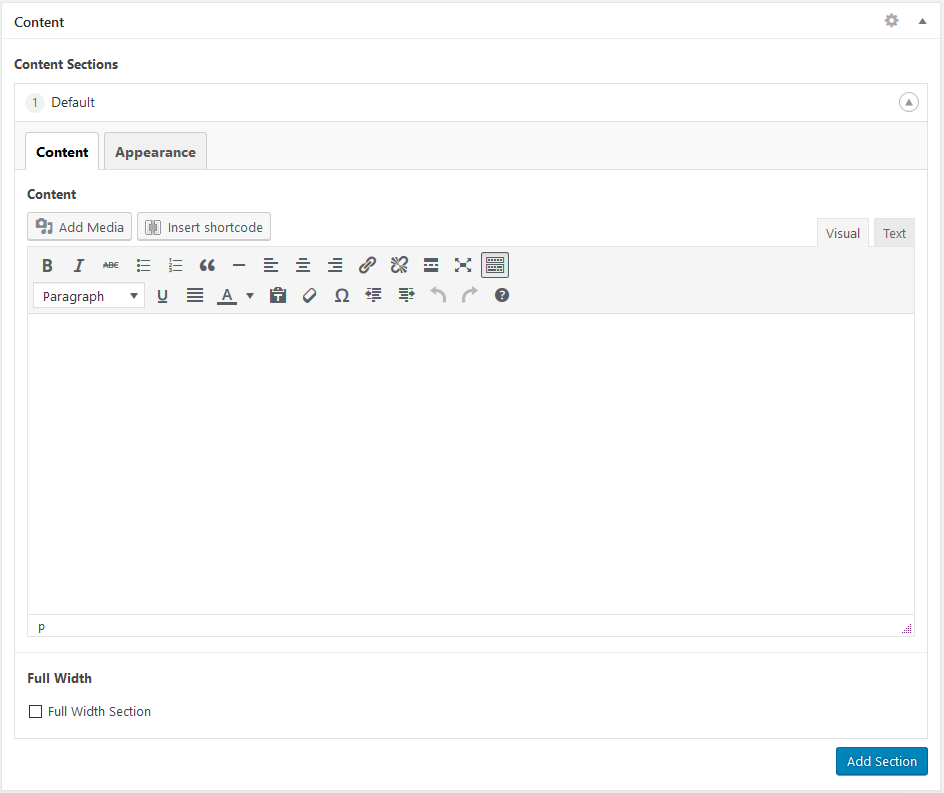
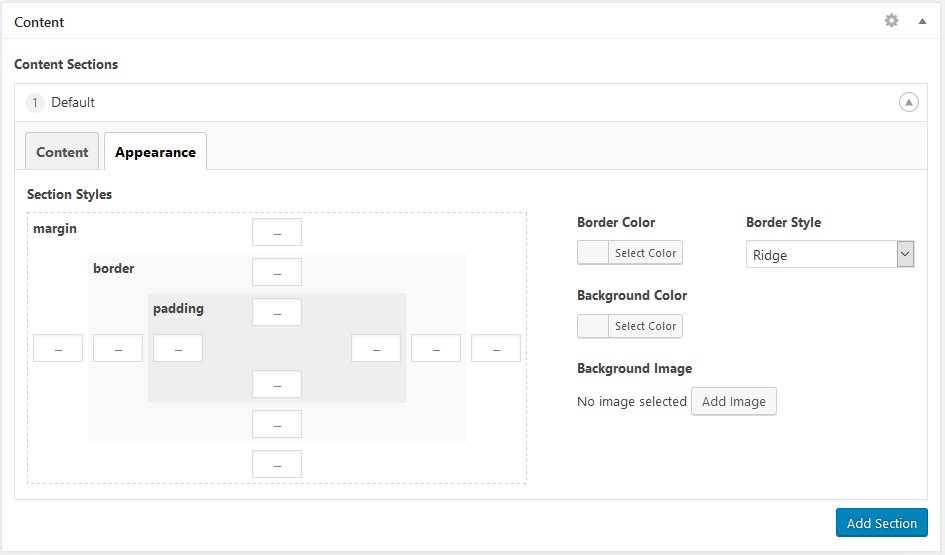
Displaying Your Content
To display the content you’ll add the following to the post or page template you’d like it to appear on:
if ( have_rows('content_sections') ) {
$section_id = 1;
while ( have_rows('content_sections') ) {
the_row();
$layout = get_row_layout();
echo '<section id="content-section-' . $section_id . '">';
the_sub_field( 'content' );
echo '</section>';
$section_id++;
}
}
One thing to note is that each section is getting a unique id corresponding to the order that it gets displayed. This is important for applying the styles to the proper sections.
Applying Styles to Content Sections
This method of using wp_add_inline_styles
assumes you are using a theme that is enqueuing its stylesheet (such as Bones or Sage).
If your theme does not enqueue its CSS (or you’d prefer to just output the styles yourself) you can also just output the styles directly using the wp_head
hook.
In your functions.php
file (or wherever your stylesheets are being enqueued) you’ll add the following:
function assets() {
wp_enqueue_style('my-css', get_stylesheet_directory_uri('styles/main.css'), false, null);
$inline_css = '';
// Section styles
if ( have_rows('content_sections') ) {
$section_id = 1;
while ( have_rows('content_sections') ) {
the_row();
$styles = get_sub_field( 'section_styles' );
// element id
$inline_css .= "
#content-section-{$section_id} {
";
// set background
if ( !empty( $styles['background_image'] ) ) {
$inline_css .= "
background-image: url({$styles['background_image']['url']});
";
}
// set other styles
$inline_css .= "
background-color: {$styles['background_color']};
border-style: {$styles['border_style']};
border-color: {$styles['border_color']};
border-width: {$styles['border_width']};
margin: {$styles['margin']};
padding: {$styles['padding']};
";
// end element id
$inline_css .= "
}
";
$section_id++;
}
}
wp_add_inline_style( 'my-css', $inline_css );
}
add_action('wp_enqueue_scripts', 'assets');
In this snippet the function assets is being called on the wp_enqueue_scripts
hook. The first line of the function enqueues the stylesheet with a handle named my-css
.
The next lines of code loop through the sections (similar to what is being done to display the sections in the template from the prior code snippet), except this time it’s looking at the section_styles field. For each section it’s appending the section specific CSS to the $inline_css
string, which uses each section’s unique id as the selector.
After all the sections have been looped through the wp_add_inline_style
function is called using the handle name that was used to enqueue the stylesheet, in this case my-css.
The result is that these inline styles will appear right after the stylesheet is included in the theme, applying the styles to the desired sections.
Using the wp_head hook
If you don’t have a theme that enqueues it’s styles, or just want to output the styles in the head section directly, you could modify the code:
function assets() {
wp_enqueue_style('my-css', get_stylesheet_directory_uri('styles/main.css'), false, null);
$inline_css = '';
// Section styles
if ( have_rows('content_sections') ) {
$section_id = 1;
while ( have_rows('content_sections') ) {
the_row();
$styles = get_sub_field( 'section_styles' );
// element id
$inline_css .= "
#content-section-{$section_id} {
";
// set background
if ( !empty( $styles['background_image'] ) ) {
$inline_css .= "
background-image: url({$styles['background_image']['url']});
";
}
// set other styles
$inline_css .= "
background-color: {$styles['background_color']};
border-style: {$styles['border_style']};
border-color: {$styles['border_color']};
border-width: {$styles['border_width']};
margin: {$styles['margin']};
padding: {$styles['padding']};
";
// end element id
$inline_css .= "
}
";
$section_id++;
}
}
echo '<style>' . $inline_css . '</style>';
}
add_action('wp_head', 'assets');